I saw this RFID kit on Thinkgeek and I was like awesome! I want that! Well it took me a few months but I eventually bought it. I was messing around, and what's really cool about it is that the company, Phidgets, supplies code samples in several languages for all platforms. So at first I was writing a reader in C# on windows, then I switched to linux and (after looking at the C code and remembering that C was bloody annoying to write) had it running in python.
Which is not to say the kit is perfect. I was disappointed by a few aspects of that ended up limiting my ideas for what to do with it...
- The reader is tuned for only the EM4102 protocol. I'm not an RFID expert, but the practicality of this is that it couldn't read my Smartcard (the PATH train between NJ and NY) or my work access card.
- The reader is limited to 3 inches. So I can't scan everything exiting a door for example.
- The reader is USB, which apparently degrades unusably after 15 feet. (The book uses a USB to Ethernet to Wireless system to extend range)
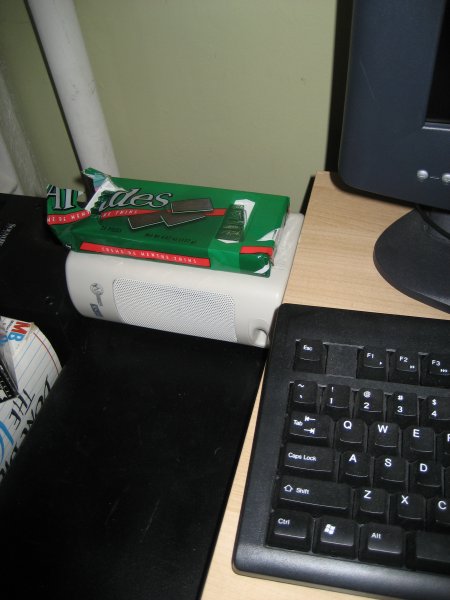
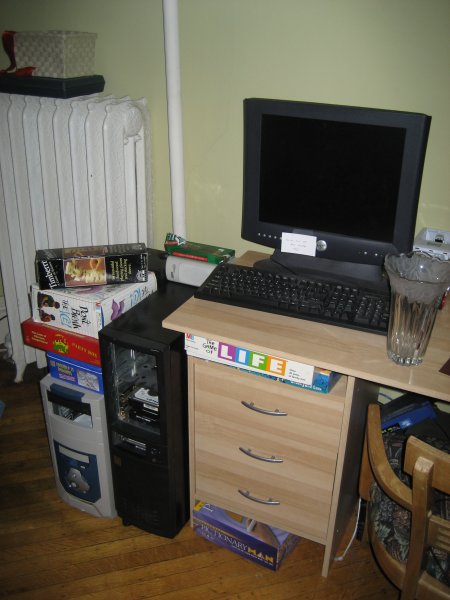
#!/bin/bash function printline { for i in `seq 1 $1`; do for j in `seq 1 80`; do echo -n " " > /dev/tty0 done done } function clearcolor { setterm -term linux -background $1 > /dev/tty0 printline 30 } function print { setterm -reset > /dev/tty0 printline 9 ./figlet-mod -f banner $1 > /dev/tty0 } setterm -blank 0 -term linux > /dev/tty0 setterm -reset > /dev/tty0 for i in `seq 1 4`; do clearcolor red clearcolor yellow done clearcolor black print "Excuse Me" sleep 6 print "Please put that back" sleep 6 print "My candy." sleep 3 print "I'm waiting."
There's two things I need to note about this code:
- I struggled a lot with a way to get the monitor to "wake up" from standby (blank screen) mode and in general do the stuff I wanted. setterm piped to the console tty eventually did the trick but figuring that out and playing around with it took a little bit.
-
figlet-mod was a modification of figlet a program similar to, but more powerful than, banner. I had to modify it becase, on a terminal sitting on the login screen, when you print some characters and then a newline, it doesn't reset the cursor position to the beginning of the line! It sits in the middle! So I ended up removing the newline it printed and instead just printing spaces until it wrapped. That was another really annoying struggle.
And what's more, I did this before I messed with setterm. And setterm has options relating to line wrapping and cursor position. So I probably could have completely avoided this. But I already did it so I ignored those setterm options. Anyway, here's the figlet diff:
diff -C 2 figlet222/figlet.c orig/figlet222/figlet.c *** figlet222/figlet.c Sat Mar 21 19:28:35 2009 --- orig/figlet222/figlet.c Tue Jul 5 05:50:49 2005 *************** *** 1476,1482 **** char *string; { ! int i,len,total; - total=80; len = MYSTRLEN(string); if (outputwidth>1) { --- 1476,1481 ---- char *string; { ! int i,len; len = MYSTRLEN(string); if (outputwidth>1) { *************** *** 1490,1500 **** } } - for (i=0;i<len;i++) { putchar(string[i]==hardblank?' ':string[i]); } ! for(;i<total;i++) { ! putchar(' '); ! } } --- 1489,1496 ---- } } for (i=0;i<len;i++) { putchar(string[i]==hardblank?' ':string[i]); } ! putchar('\n'); }
The script it ran when you put the tag back. It includes all the functions from before, just omitted for brevity.
setterm -blank 0 -term linux > /dev/tty0 setterm -reset > /dev/tty0 for i in `seq 1 2`; do clearcolor green clearcolor blue done clearcolor black killall freak.sh print "Thank you" sleep 10 clearcolor black setterm -blank 1 -term linux > /dev/tty0
This code is just a stripped down, modified version of what it freely available from the Phidgets website. I'm including it for completeness, but all I did was rip out print statements and call the shell scripts.
#!/usr/bin/env python from ctypes import * import sys import os from Phidgets.PhidgetException import * from Phidgets.Events.Events import * from Phidgets.Devices.RFID import RFID rfid = RFID() def rfidError(e): print "Phidget Error %i: %s" % (e.eCode, e.description) return 0 def rfidTagGained(e): rfid.setLEDOn(0) os.system("./thankyou.sh") return 0 def rfidTagLost(e): rfid.setLEDOn(1) os.system("./freak.sh") return 0 #Main Program Code try: rfid.setOnErrorhandler(rfidError) rfid.setOnTagHandler(rfidTagGained) rfid.setOnTagLostHandler(rfidTagLost) except PhidgetException, e: print "Phidget Exception %i: %s" % (e.code, e.message) exit(1) try: rfid.openPhidget() except PhidgetException, e: print "Phidget Exception %i: %s" % (e.code, e.message) exit(1) try: rfid.waitForAttach(10000) except PhidgetException, e: print "Phidget Exception %i: %s" % (e.code, e.message) try: rfid.closePhidget() except PhidgetException, e: print "Phidget Exception %i: %s" % (e.code, e.message) exit(1) exit(1) rfid.setAntennaOn(True) chr = sys.stdin.read(1) try: rfid.closePhidget() except PhidgetException, e: print "Phidget Exception %i: %s" % (e.code, e.message) exit(1) exit(0)
required, hidden, gravatared
required, markdown enabled (help)
* item 2
* item 3
are treated like code:
if 1 * 2 < 3:
print "hello, world!"
are treated like code: